Hint: The majority of the time was spent on the art. Here’s the game I made in it, playable in browser.
Tools:
- Ren’Py (free to download!)
- Art (a character base and clothes)
Screens.rpy
default mtop = 0 ## Define each of the variables you use later
screen dressup():
tag menu
## This adds a background to the screen- in my case, I added a beach background
add "dressup bg"
## This is the character base! The transform is defined in script.rpy
add "base.png" at items
Script.rpy
## Define a position for the base and items as a transform so if you need to change it
## later you only have to change this!
transform items:
xalign 0.0
yalign 0.05
label start:
## This calls the dressup screen from screens.rpy
call screen dressup
Now we’re going to start coding the top textbutton which will let us cycle through the shirt options in screens.rpy. NOTE: Please name your images in a pattern, such as “top1”, “top2”, “top3”, etc. It makes it SO much easier!
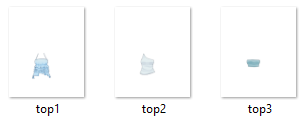
Screens.rpy
## This is the button "Top" which, when toggled, cycles through the tops available.
## The "mtop" inside the SetVariable should be whatever variable you decide to use
## for this item. Change the number after the % to however many clothing items you have.
textbutton "Top" action SetVariable("mtop", (mtop) % 3 + 1) xalign 0.86 yalign 0.12:
## This text here is more for polish- i.e., it's to make the button look good, but
## the button will still work without it. Remember to use a colon after the first line
## if you follow it up with text like this that's indented.
text_size 36 ## Changes text size
text_color "#fff" ## Changes text color
text_hover_color "#6ba0e3" ## Changes text hover color
text_outlines [ (2, "#0049a8", 0, 0) ] ## Adds an outline the text
## This textbutton basically resets the Top button by setting it to a default "0"
## as you can see in the SetVariable
textbutton "x" action SetVariable("mtop", 0) xalign 0.90 yalign 0.125:
text_size 24
text_color "#d5dee8"
text_hover_color "#6ba0e3"
text_outlines [ (2, "#0049a8", 0, 0) ]
## NOTE: All of this should be indented under the screen dressup!
Now we need to actually show the tops! We can do this multiple ways- I’ll go over the simpler way first.
Screens.rpy
## This is a simple if statement! Basically, if the variable is equal to 1, then it'll
## display the image called "top1" that's in our images folder at the transform we
## defined earlier. Increase this according to however many tops you have.
if mtop == 1:
add "top1" at items
elif mtop == 2:
add "top2" at items
elif mtop == 3:
add "top3" at items
Here’s a more complicated but time-saving way:
Screens.rpy
## This goes right under the textbuttons at the same level of indentation.
## Basically, it adds an image "top(variable number)" and inputs whatever
## number the "mtop" is at in the {} section.
add "top{}".format(mtop)
Here’s a bigger example of what it all should look like (with both examples of how to display the images):
Screens.rpy
default mtop = 0
screen dressup():
tag menu
add "dressup bg"
add "base.png" at items
textbutton "Top" action SetVariable("mtop", (mtop) % 14 + 1) xalign 0.86 yalign 0.12:
text_size 36
text_color "#fff"
text_hover_color "#6ba0e3"
text_outlines [ (2, "#0049a8", 0, 0) ]
textbutton "x" action SetVariable("mtop", 0) xalign 0.90 yalign 0.125:
text_size 24
text_color "#d5dee8"
text_hover_color "#6ba0e3"
text_outlines [ (2, "#0049a8", 0, 0) ]
## If you're using the if statements for the images
if mtop == 1:
add "top1" at items
elif mtop == 2:
add "top2" at items
elif mtop == 3:
add "top3" at items
## If you're using the format option for showing images- NOTE: don't use both the if
## statement above and this!
add "top{}".format(mtop)
And that’s pretty much the basics for making a dress-up game in Ren’Py! Remember that when you’re showing the images that the further down on the screen they are, the more “on top” they’ll be ingame- i.e., we put the background as the very first image added on the screen since it needed to be the furthest away one.
There’s still a lot of things you can add such as an About button for the About menu, a music slider, etc, so I’ll go over a couple of those here as well.
Screens.rpy
## This adds an "About" button to the screen that sends people to the About menu that is
## already made in the New GUI for Ren'Py.
textbutton "About" action ShowMenu("about") xalign 0.98 yalign 0.0:
text_size 22
text_color "#fff"
text_hover_color "#6ba0e3"
text_outlines [ (2, "#0049a8", 0, 0) ]
## This adds a music volume slider to the game! I added music by selecting a piece to play
## on the main menu (which can be added in gui.rpy) and it auto plays in the full game.
vbox:
xalign 0.975
yalign 0.85
## This just adds a title that says "Music Volume" so people know what the
## bar below it is for.
label _("Music Volume"):
text_size 30
text_color "#fff"
text_outlines [ (2, "#0049a8", 0, 0) ]
xalign 0.97
## This is the slideable bar that changes the music volume.
bar value Preference("music volume") xsize 230
## The "xsize" changes how wide the bar is.
If you have any questions or want to share progress, feel free to join the Devtalk+ discord server! Also feel free to @ me on Twitter with any questions or completed games! ♥